Gravity Sim Job System - Video 01 - Overview and GravityComponent
YouTube Video #1 - Gravity Series
First video in the Gravity Sim Job System Series.
In this video we discuss some math behind gravity and the software design we intend to build. This will be a completely independent physics system and any GameObjects to participate in this should have their colliders removed for maximum performance.
We then write a GravityComponent
structure and a GravityBehaviour
MonoBehaviour so we can use the Inspector to set different initial values on GameObjects in scene or that are to be used as prefabs. The code for these are at the bottom of this page.
This series will demonstrate how to use the C# Job System and Unity Mathematics to build a mini ECS software design that evolves over a series of videos.
Gravity Calculation
The first step is to calculate the force applied due to gravity.
This will be one job that takes GravityComponent
as input and generates a sum of forces vector.
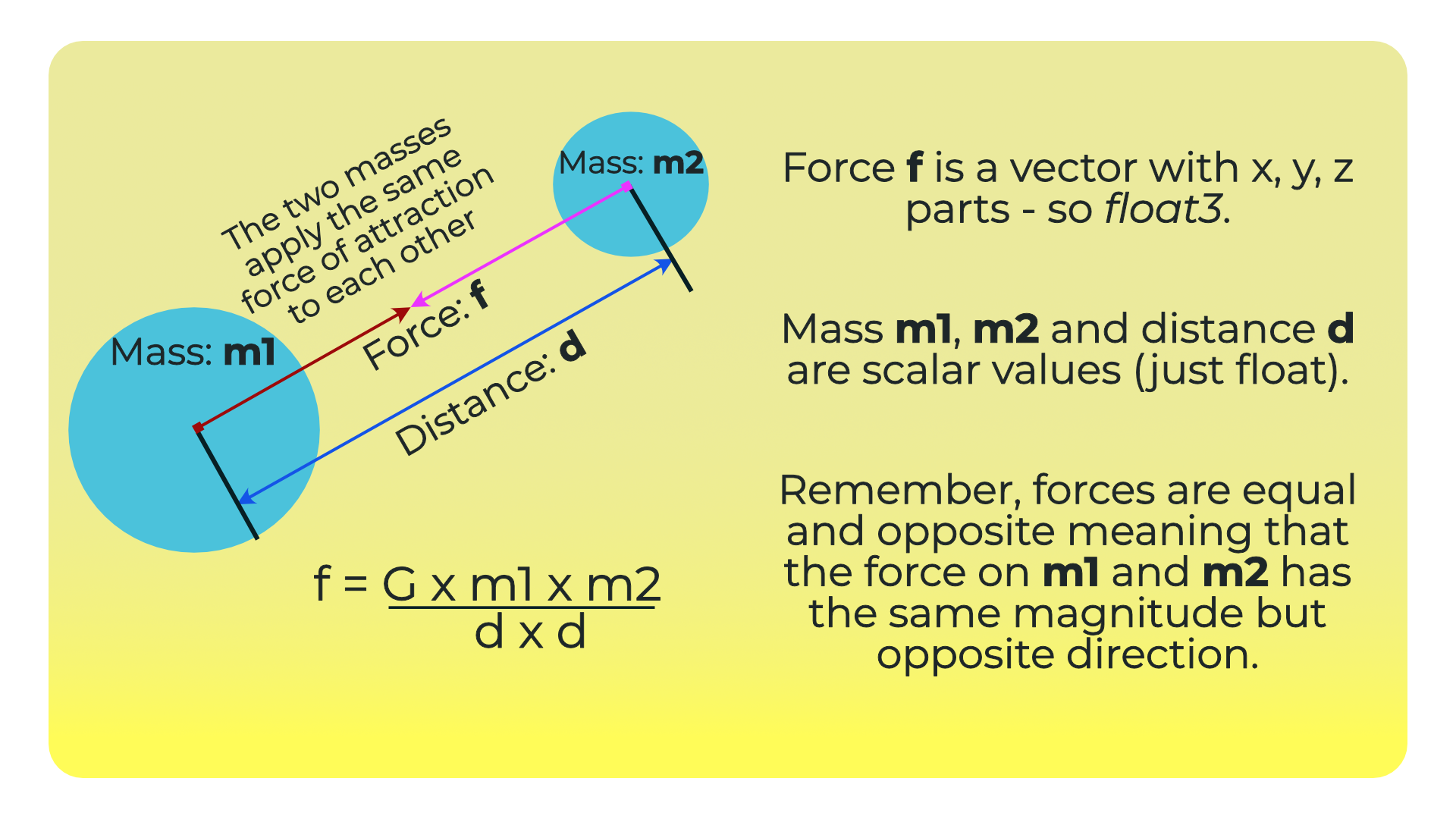
From this, the acceleration, velocity and position can be computed. This set of computations could be done in a single job or a pipeline of jobs.
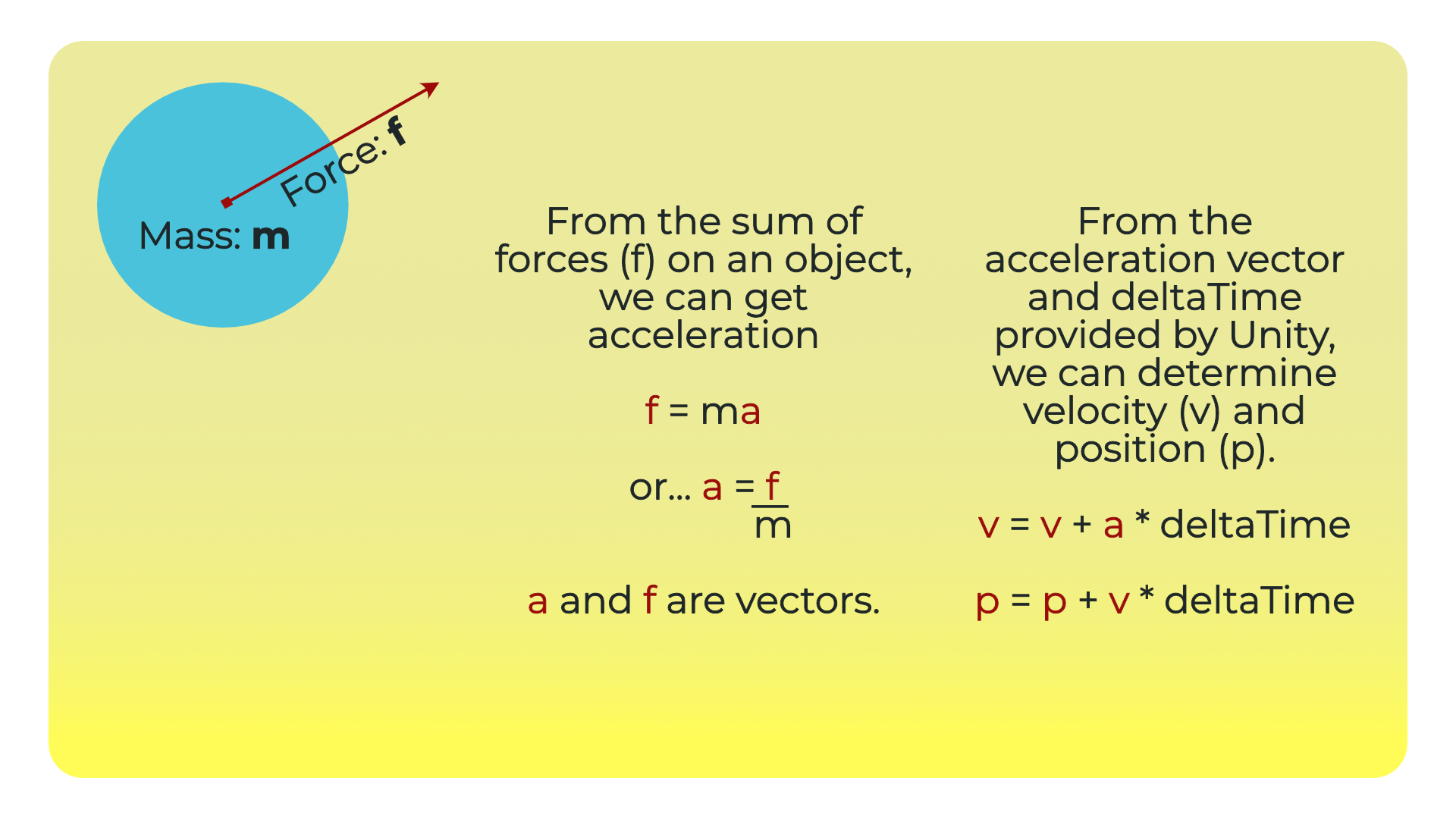
Mini ECS Design
The code will be organised into components, jobs and a system class to organise the jobs and manage the arrays.
The system class will be managed by the GravityController
MonoBehaviour that enables gravity in the scene.
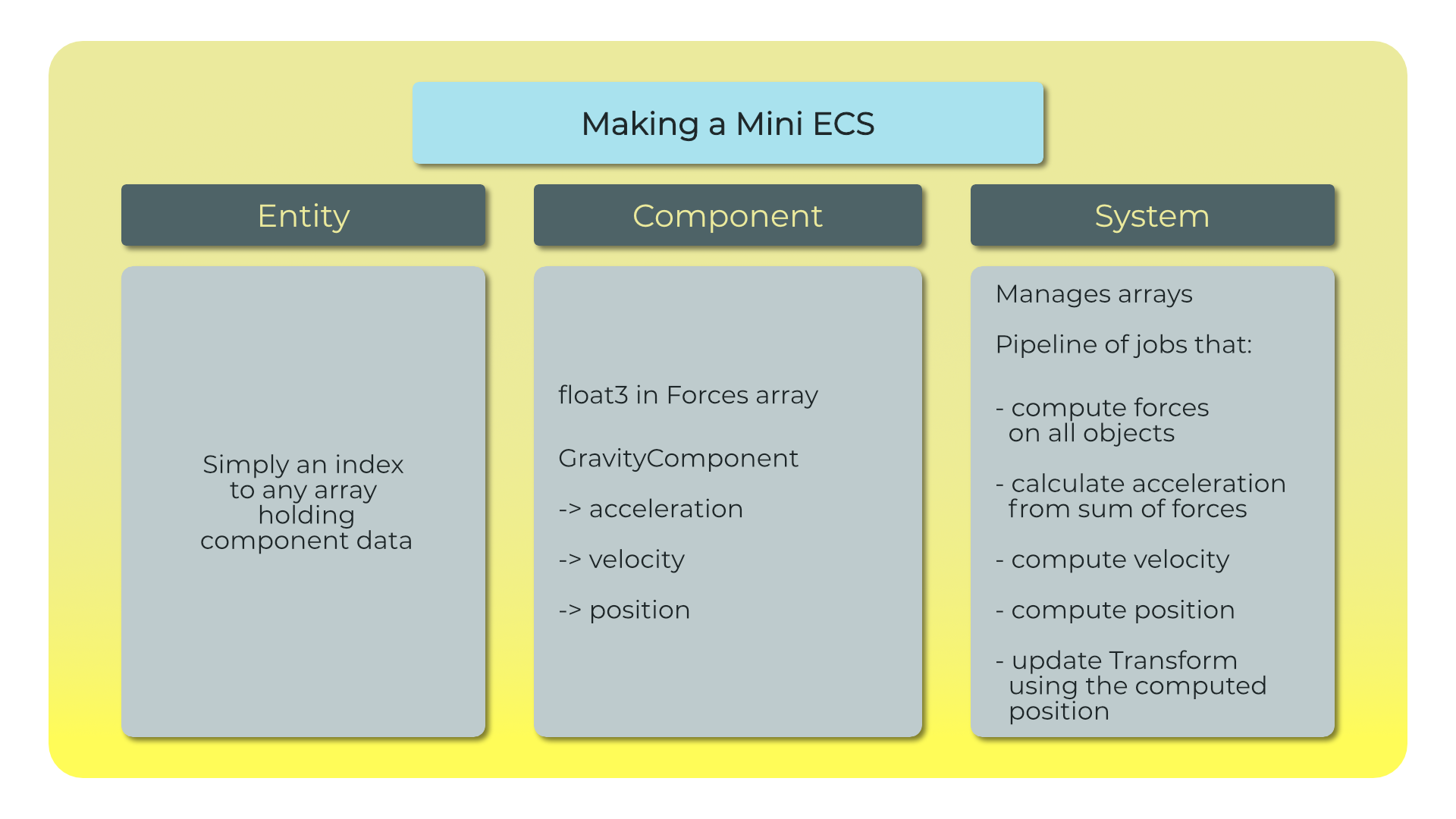
Code Written in this Video
The component which is a value object that is stored, and initialized, by the GravityBehaviour.
GravityComponent.cs
using Unity.Mathematics;
[System.Serializable]
public struct GravityComponent
{
public float3 Position;
public float3 Velocity;
public float3 Acceleration;
public float Mass;
}
GravityBehaviour.cs
using UnityEngine;
[AddComponentMenu("DesignerTech/Gravity Settings")]
public class GravityBehaviour : MonoBehaviour
{
[Tooltip("Set the gravity parameters for this prefab")]
public GravityComponent GravitySettings;
}